Getting Started With Ethers.js Ethereum Development: Your 2024 Guide
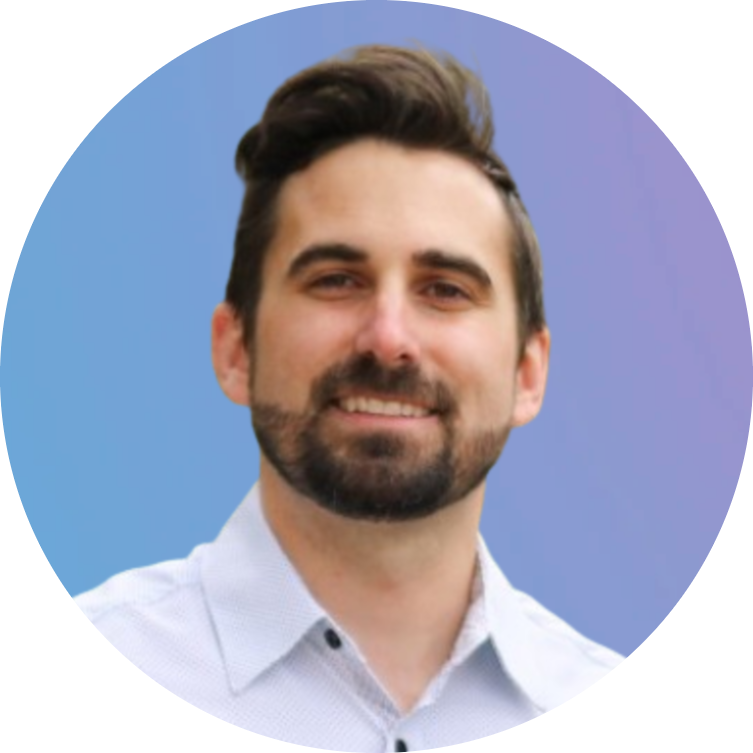
Kevin Dwyer
September 16, 2024
5 min read
Ethereum, the network that revolutionized blockchain technology with smart contracts and decentralized applications (dApps), is a thriving ecosystem of innovation now more than ever. If you're a developer excited to join the Ethereum community, you'll need the right tools. Ethers.js is the premier JavaScript library that unlocks the full potential of Ethereum development. In this comprehensive guide, we'll dive deep into ethers.js, exploring its capabilities, best practices, and real-world applications.
Access the free Ethereum RPC now →
Why ethers.js Reigns Supreme for Ethereum Development
Ethers.js has become the go-to library for Ethereum developers for compelling reasons:
- Unparalleled Developer Experience: ethers.js boasts a clear, concise syntax, intuitive APIs, and comprehensive documentation. Whether you're a beginner or a seasoned pro, you'll find it remarkably easy to use.
- Lightweight and Performant: Built with efficiency in mind, ethers.js is laser-focused on core Ethereum functionality. This makes it a nimble and responsive choice for your dApp projects.
- Complete Arsenal of Features: From connecting to nodes and managing wallets to interacting with smart contracts and handling events, ethers.js equips you with everything you need to build sophisticated dApps.
- Thriving Community and Ecosystem: ethers.js enjoys widespread adoption, backed by a vibrant community of developers, extensive tutorials, and a wealth of resources.
Kickstart Your Ethereum Journey with ethers.js
Be sure to check out Ethers.js for the latest documentation version.
Installation
npm (Preferred): Install ethers.js through npm, the Node Package Manager, for seamless integration with your Node.js projects:
# Install ethers.js
npm install ethers
Everything in Ethers can be accessed either directly from the main library or through the ethers object. The package.json file also includes options that let you import specific parts of the library if needed.
importing in Node.js
// Import everything
import { ethers } from "ethers";
// Import just a few select items
import { BrowserProvider, parseUnits } from "ethers";
// Import from a specific export
import { HDNodeWallet } from "ethers/wallet";
Importing ESM in a browser
<script type="module">
import { ethers } from "https://cdnjs.cloudflare.com/ajax/libs/ethers/6.7.0/ethers.min.js";
// Your code here...
</script>
Setting Up Your Development Sanctuary
- Code Editor: Choose your weapon wisely. Visual Studio Code, Atom, or Sublime Text are excellent options for JavaScript development.
- Node.js and npm: Ensure you have Node.js and npm installed to run JavaScript code and manage packages.
- Ethereum Node: You'll need a gateway to the Ethereum blockchain. Run your own node or leverage services like Ankr’s Free Node API for Ethereum.
Grasp the Core ethers.js Concepts
- Providers: Your bridge to the Ethereum network. Providers enable you to fetch blockchain data, send transactions, and interact with smart contracts.
- Signers: The embodiment of Ethereum accounts. Signers allow you to sign transactions, messages, and authorize operations on the blockchain.
- Contracts: ethers.js provides powerful classes to seamlessly interact with smart contracts. Read data, execute functions, and listen for events effortlessly.
Seamlessly Connect to Ethereum - Your Options for Node Connections
- Ankr or another provider: Ankr offers an RPC/API solution that provides easy access to Ethereum nodes with various subscription tiers.
- Self-Hosted Node: Take complete control of your node infrastructure, but be prepared for technical overhead.
- Local Development Networks: Ganache and Hardhat allow you to simulate local Ethereum environments for testing and development.
Ethers.js MetaMask Integration Made Easy
Ethers.js seamlessly integrates with MetaMask, the popular browser wallet. Here's a simplified example:
import { ethers } from "ethers";
async function connectToMetaMask() {
const provider = new ethers.providers.Web3Provider(window.ethereum);
await provider.send("eth_requestAccounts", []);
const signer = provider.getSigner();
// Your 'signer' is ready to interact with the blockchain!
}
Connect with Other Wallets (ethers js connect wallet)
Ethers.js extends its support to other wallets like WalletConnect, Coinbase Wallet, and many more. Check out the official documentation for detailed instructions.
Mastering Smart Contract Interactions (ethers js connect to contract)
Reading Contract Data: Unveiling the Secret
const contract = new ethers.Contract(contractAddress, contractABI, provider);
const balance = await contract.balanceOf(address);
console.log(`Balance of ${address}: ${balance}`);
Writing to Smart Contracts: Making Your Mark
const tx = await contract.connect(signer).transfer(toAddress, amount);
await tx.wait();
console.log("Transaction successful!", tx.hash);
Listening for Events: Stay Informed
contract.on("Transfer", (from, to, amount, event) => {
console.log(`${from} sent ${amount} to ${to}`);
});
Beyond the Basics: Advanced ethers.js Techniques & Use Cases
Gas Estimation and Optimization
Optimize transaction costs by accurately estimating gas fees and applying optimization strategies.
Error Handling and Security: Building Robust dApps
Protect your dApps by implementing comprehensive error handling and adhering to security best practices.
Unleash Your Creativity: Build with ethers.js
ethers.js is your gateway to building incredible Ethereum applications:
- Decentralized Finance (DeFi): Create lending platforms, decentralized exchanges (DEXs), yield farming protocols, and more.
- Non-Fungible Tokens (NFTs): Design marketplaces, minting platforms, and unique digital assets.
- Decentralized Autonomous Organizations (DAOs): Build governance systems, voting mechanisms, and community-driven organizations.
- Gaming and Metaverse: Develop play-to-earn games, virtual worlds, and interactive experiences.
Additional Resources: Your Path to Mastery
Official ethers.js Documentation: Your comprehensive resource for everything ethers.js. ethers.js GitHub Repository: Explore the source code, report issues, and contribute to the project. Community Forums and Discord: Connect with fellow developers, seek help, and share your knowledge. Online Courses and Tutorials: Numerous online resources offer in-depth ethers.js tutorials and project-based learning.
Ready to embark on your Ethereum development journey?
With ethers.js as your trusted companion, the possibilities are endless. Dive in, experiment, and unleash your creativity in the world of decentralized applications.
Join the conversation on our channels!
Twitter | Telegram Announcements | Telegram English Chat | Help Desk | Discord | YouTube | LinkedIn | Instagram | Ankr Staking
Similar articles.
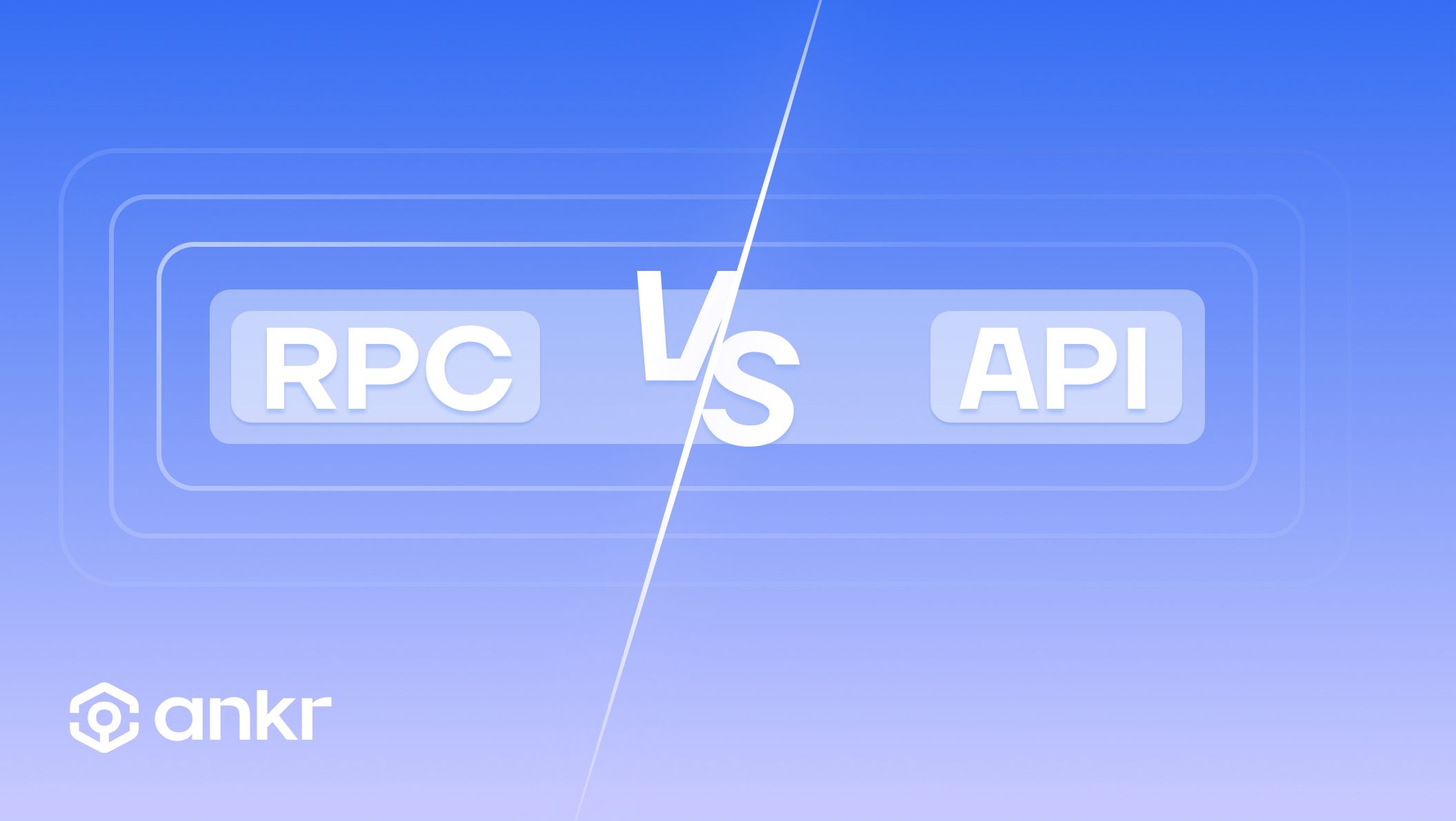
What's the Difference Between RPCs and APIs?
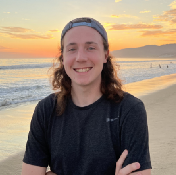
Ethan Nelson
December 15, 2022
If you are just beginning your journey into the world of Web3 development, you may have come across terms such as "RPC" and "API" and...
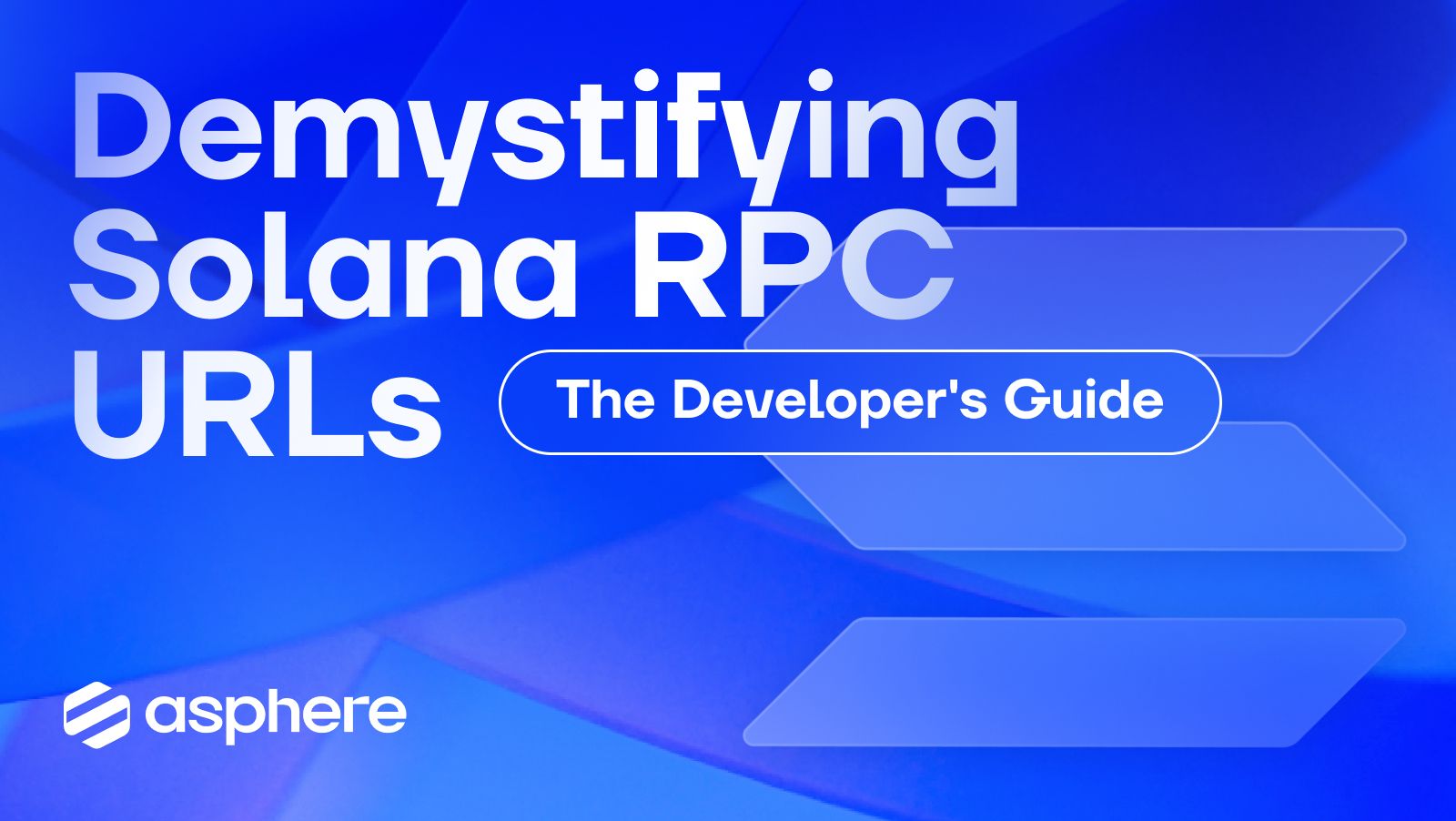
Solana RPC URLs: Guide for Developers and Users + Solana Chain IDs
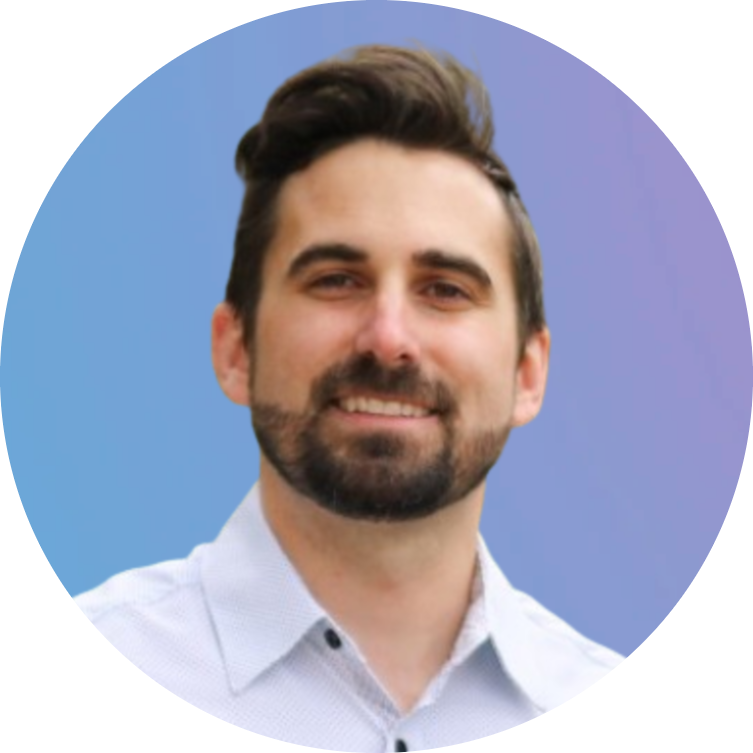
Kevin Dwyer
August 30, 2024
As a developer diving into the Solana ecosystem, understanding Remote Procedure Call (RPC) URLs is crucial. These URLs serve as your gateway to interact with...
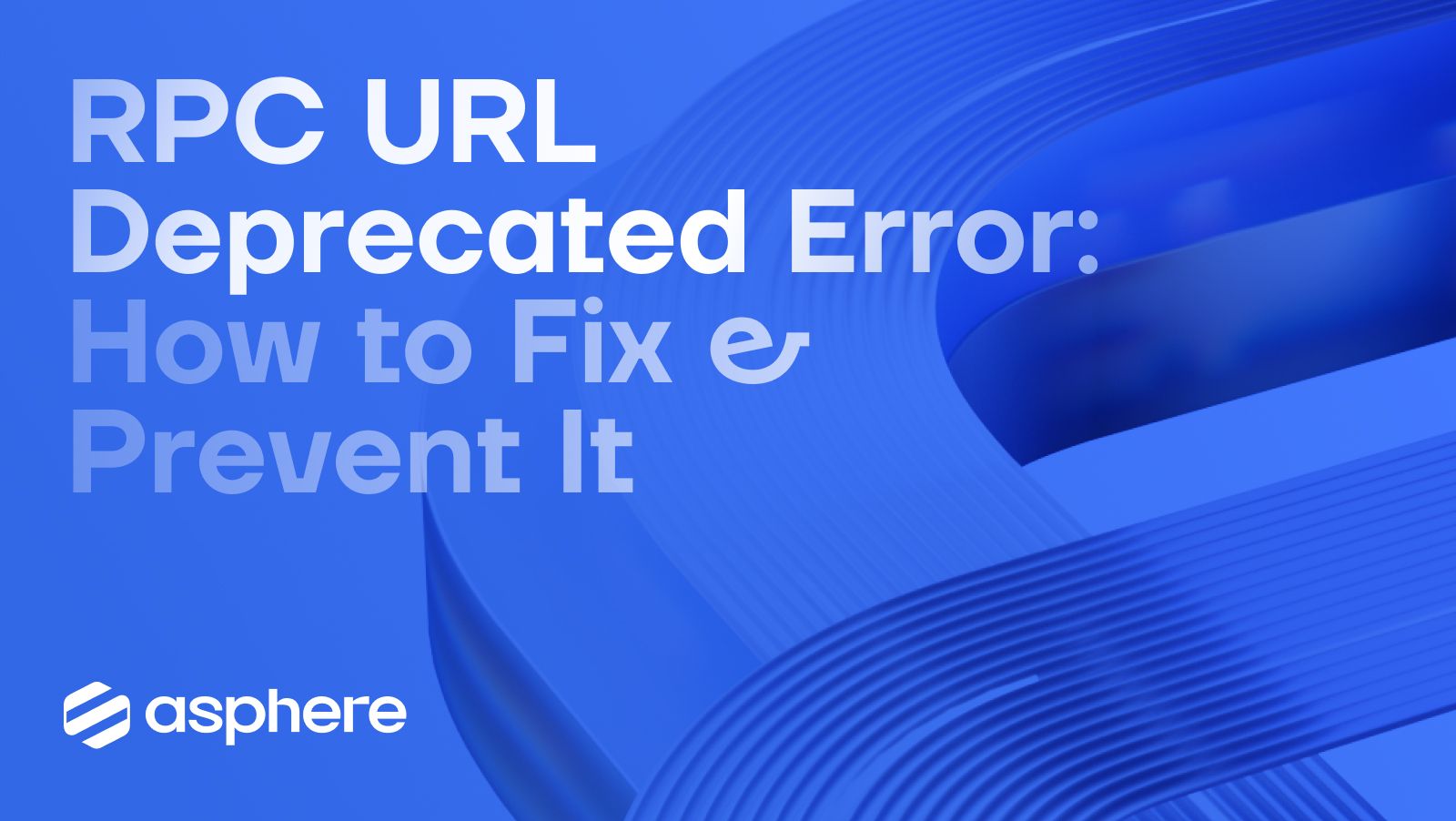
RPC URL Deprecated Error: How to Fix & Prevent It (2024 Guide)
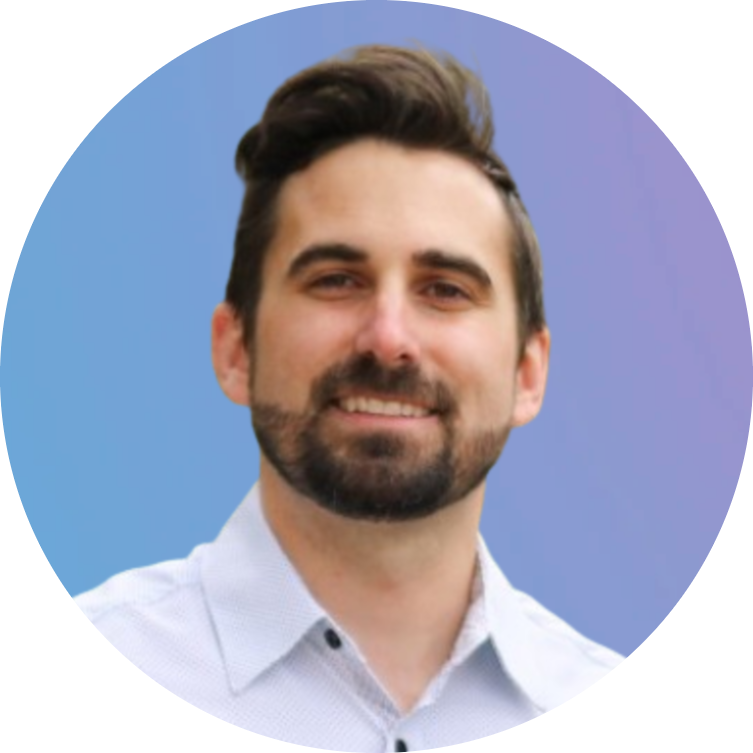
Kevin Dwyer
September 5, 2024
If you've ever encountered the dreaded "RPC URL Deprecated" error while working with cryptocurrency wallets or decentralized applications (dApps), you're not alone. This error message,...